🎈 What is Notification
-
Notification
is a way to display messages on the desktop after the browser is minimized - Similar to
360
and other rogue software pop-up advertisements in the lower right corner of the desktop - It is detached from the browser, and the message is on the top

🎈 Pop-up authorization
- Authorize the current page to allow notifications
- You can see if you already have permission by checking the value of the read-only attribute
Notification.permission
- default: the user has not been asked whether to authorize, you can pass
Notification.requestPermission()
to ask the user whether to allow notifications - granted: the state after the user clicks to allow
- denied: the state after the user clicks to deny, the notification box is unavailable
Notification.requestPermission()
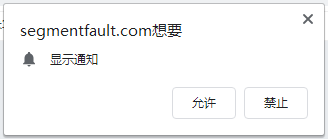
🎈 Popup usage
- You can use the notification push function through
new Notification($title, $options)
- title: The title of the notification that must be displayed
- options: optional, an object that is allowed to set notifications. It contains the following properties:
- dir: the direction of the text; its value can be auto (auto), ltr (left-to-right), or rtl (right-to-left)
- lang: Specifies the language used in the notification.
- body: a string to display additionally in the notification
- tag: Give the notification an ID so that the notification can be refreshed, replaced, or removed when necessary.
- icon: The URL of an image that will be used to display the notification icon.
new Notification("温馨提醒", {
body: "飞兔小哥送你一份奖品待领取",
icon: "https://autofelix.github.io/autofelix/u/favicon.ico",
data: "https://autofelix.blog.csdn.net/"
});
🎈 Browser support detection
- Before using the notification push function, you need to check whether the browser supports it
- It can be detected by the
"Notification" in window
method - On the premise of browser support, determine whether the user authorizes the notification. If not, an authorization box can pop up.
- If the user has already declined, we don't bother the user
function notify() {
// 先检查浏览器是否支持
if (!("Notification" in window)) {
alert("This browser does not support desktop notification");
}
// 检查用户是否同意接受通知
else if (Notification.permission === "granted") {
// If it's okay let's create a notification
var notification = new Notification("Hi there!");
}
// 否则我们需要向用户获取权限
else if (Notification.permission !== "denied") {
Notification.requestPermission().then(function (permission) {
// 如果用户接受权限,我们就可以发起一条消息
if (permission === "granted") {
var notification = new Notification("Hi there!");
}
});
}
// 最后,如果执行到这里,说明用户已经拒绝对相关通知进行授权
// 出于尊重,我们不应该再打扰他们了
}
🎈 Authorization callback
- The notification has four callback methods
- onshow: called when the notification is displayed
- onclose: called when the notification is closed
- onclick: called when the notification is clicked
- onerror: called when a notification error occurs
var notification = new Notification("温馨提醒", {
body: "飞兔小哥送你一份奖品待领取",
icon: "https://autofelix.github.io/autofelix/u/favicon.ico",
data: "https://autofelix.blog.csdn.net/"
});
notification.onshow = function (event) {
console.log("show : ", event);
};
notification.onclose = function (event) {
console.log("close : ", event);
};
notification.onclick = function (event) {
console.log("click : ", event);
// 当点击事件触发,打开指定的url
window.open(event.target.data)
notification.close();
};
notification.onerror = function (event) {
console.log("close : ", event);
};
🎈 Close the popup after 3 seconds
- Implement the function of closing the pop-up window after 3 seconds
var notification = new Notification('标题');
notification.onshow = function () {
setTimeout(function () {
notification.close();
}, 3000);
}
**粗体** _斜体_ [链接](http://example.com) `代码` - 列表 > 引用
。你还可以使用@
来通知其他用户。